// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
interface IERC721 {
function ownerOf(uint256 tokenId) external view returns (address);
function transferFrom(address from, address to, uint256 tokenId) external;
}
/// @title NFT Ownership Verification and Secure Transfer Contract
/// @dev Enables verification and secure peer-to-peer transfers
contract NFTOwnershipVerifier {
event OwnershipVerified(address indexed user, uint256 indexed tokenId);
event NFTTransferred(address indexed from, address indexed to, uint256 indexed tokenId);
/// @notice Verifies if a user owns a specific NFT
/// @param nftContract Address of the ERC-721 contract
/// @param tokenId ID of the NFT
/// @param user Address of the potential owner
/// @return bool Whether the user owns the token
function verifyOwnership(address nftContract, uint256 tokenId, address user)
external
view
returns (bool)
{
IERC721 nft = IERC721(nftContract);
bool isOwner = nft.ownerOf(tokenId) == user;
if (isOwner) {
emit OwnershipVerified(user, tokenId);
}
return isOwner;
}
/// @notice Securely transfers an NFT between two users
/// @param nftContract Address of the ERC-721 contract
/// @param tokenId ID of the NFT
/// @param from Current owner of the NFT
/// @param to New recipient of the NFT
function secureTransfer(
address nftContract,
uint256 tokenId,
address from,
address to
) external {
IERC721 nft = IERC721(nftContract);
require(nft.ownerOf(tokenId) == from, "Sender does not own the NFT");
nft.transferFrom(from, to, tokenId);
emit NFTTransferred(from, to, tokenId);
}
}
Own the web. Build without limits.
Decentralized. Trustless. Borderless. Create the future of the internet with cutting-edge Web3 technology—where you’re in control.
Build smarter . Scale seamlessly .
Effortlessly integrate dApps, track on-chain data, and automate insights with powerful Web3-native solutions. The future is decentralized—build with confidence.
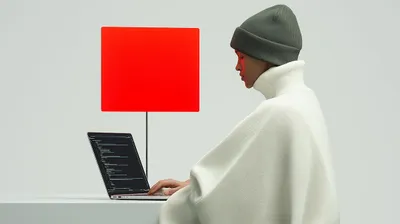
Automated Transactions
Schedule and execute blockchain transactions with precision using trustless automation and smart contracts.
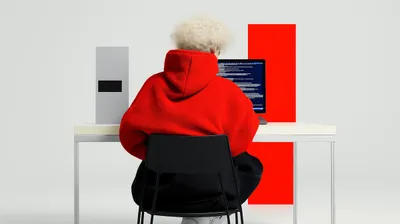
Self-Executing Smart Contracts
Automate complex transactions with self-executing smart contracts, ensuring seamless, tamper-proof, and permissionless operations on the blockchain.
Immutable Transactions
Every transaction is permanently recorded on-chain, ensuring transparency, security, and trustless execution without intermediaries.
Gas-Efficient Smart Contracts
Optimize on-chain interactions with low-cost, gas-efficient smart contracts designed for speed, security, and seamless interoperability.
Build smarter Scale seamlessly
Effortlessly integrate dApps, track on-chain data, and automate insights with powerful Web3-native solutions. The future is decentralized—build with confidence.
-
Seamless Web3 Integration
-
Effortlessly connect your dApps, wallets, and smart contracts with our plug-and-play solutions.
-
Customizable Smart Contracts
-
Easily deploy and manage tailor-made smart contracts for your decentralized applications.
-
On-Chain Data Tracking
-
Monitor real-time blockchain transactions and track assets across multiple networks.
-
Automated Blockchain Analytics
-
Generate real-time reports on token flows, smart contract activity, and user engagement.
Build smarter. Scale seamlessly.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
/// @title Web3 Ownership Smart Contract
/// @dev Enables ownership management and secure asset transfers
contract Web3Ownership {
address public owner;
mapping(address => bool) public authorizedUsers;
event OwnershipTransferred(address indexed previousOwner, address indexed newOwner);
event UserAuthorized(address indexed user);
event UserRevoked(address indexed user);
constructor() {
owner = msg.sender;
}
/// @notice Transfers ownership to a new address
/// @param newOwner Address of the new contract owner
function transferOwnership(address newOwner) public {
require(msg.sender == owner, "Not the contract owner");
require(newOwner != address(0), "Invalid new owner");
emit OwnershipTransferred(owner, newOwner);
owner = newOwner;
}
/// @notice Authorizes a new user for specific operations
/// @param user Address to be authorized
function authorizeUser(address user) public {
require(msg.sender == owner, "Only the owner can authorize");
authorizedUsers[user] = true;
emit UserAuthorized(user);
}
/// @notice Revokes authorization from a user
/// @param user Address to be revoked
function revokeUser(address user) public {
require(msg.sender == owner, "Only the owner can revoke access");
authorizedUsers[user] = false;
emit UserRevoked(user);
}
/// @notice Checks if an address is authorized
/// @param user Address to check
function isAuthorized(address user) public view returns (bool) {
return authorizedUsers[user];
}
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
/// @title Web3 Ownership Smart Contract
/// @dev Enables ownership management and secure asset transfers
contract Web3Ownership {
address public owner;
mapping(address => bool) public authorizedUsers;
event OwnershipTransferred(address indexed previousOwner, address indexed newOwner);
event UserAuthorized(address indexed user);
event UserRevoked(address indexed user);
constructor() {
owner = msg.sender;
}
/// @notice Transfers ownership to a new address
/// @param newOwner Address of the new contract owner
function transferOwnership(address newOwner) public {
require(msg.sender == owner, "Not the contract owner");
require(newOwner != address(0), "Invalid new owner");
emit OwnershipTransferred(owner, newOwner);
owner = newOwner;
}
/// @notice Authorizes a new user for specific operations
/// @param user Address to be authorized
function authorizeUser(address user) public {
require(msg.sender == owner, "Only the owner can authorize");
authorizedUsers[user] = true;
emit UserAuthorized(user);
}
/// @notice Revokes authorization from a user
/// @param user Address to be revoked
function revokeUser(address user) public {
require(msg.sender == owner, "Only the owner can revoke access");
authorizedUsers[user] = false;
emit UserRevoked(user);
}
/// @notice Checks if an address is authorized
/// @param user Address to check
function isAuthorized(address user) public view returns (bool) {
return authorizedUsers[user];
}
}
Data that drives change, shaping the future
Decentralized, secure, and built to transform industries worldwide. See how our platform enables sustainable growth and innovation at scale.
Our platform not only drives innovation but also empowers businesses to make smarter, data-backed decisions in real time. By harnessing the power of AI and machine learning, we provide actionable insights that help companies stay ahead of the curve.
Empowering innovators, shaping the future
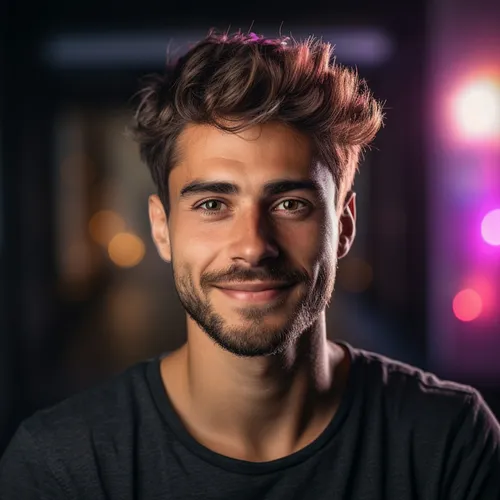
David Gutierrez
Web3 Creator & NFT Artist
"I couldn't be happier with the seamless integration. The team went above and beyond to ensure my smart contracts were optimized and secure."
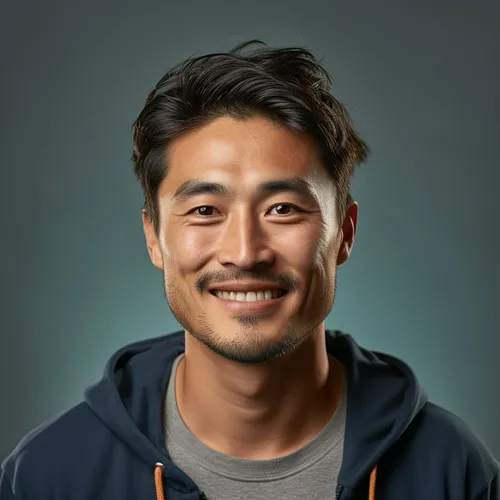
Pierluigi Camomillo
Founder of Camomille DeFi
"Their expertise in DeFi solutions is unmatched. The level of transparency and efficiency they bring to on-chain transactions is game-changing."
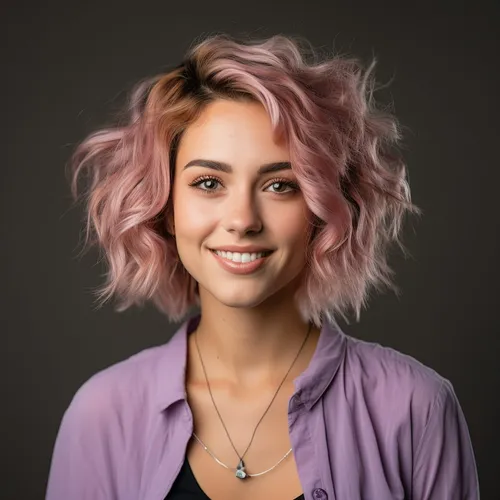
Ella Svensson
DAO Contributor & Blockchain Advocate
"The decentralization-first approach made all the difference. Finally, a team that truly understands the power of Web3!"
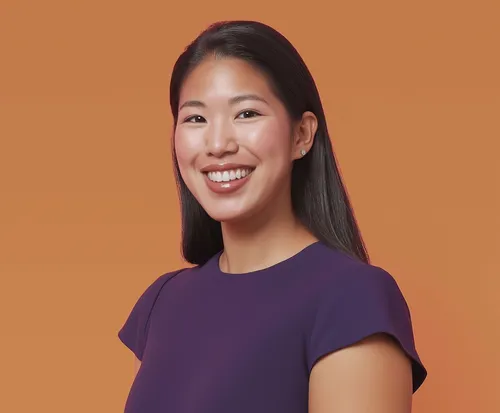
Alexa Rios
Chief Innovator at Ideatech Labs
"The personalized support and deep technical expertise helped our dApp scale effortlessly. Highly recommended for any Web3 startup!"
Easy pricing. No gas wars required.
Access all the decentralized tools you need—without breaking the bank (or your crypto stash).
Explorer
$0/month
Perfect for crypto enthusiasts.
- Deploy up to 10 smart contracts
- Access to public blockchain data
- 1 wallet integration
Builder Popular AF!
$1,999/month/month
50% off for the first 3 projects!
- Deploy 50 smart contracts
- Connect up to two dApps
- Token gated content access
- 10 API calls per second
- Multi-wallet support
Enterprise
Custom pricing/month
For large-scale Web3 applications.
- Deploy unlimited smart contracts
- Unlimited dApp integrations
- Advanced tokenomics management
- High-speed blockchain indexing
- Unlimited multi-signature wallets
Decentralized
Contact us/month
The ultimate package.
- Unlimited smart contract deployments
- Unlimited dApp integrations
- Customizable governance systems
- Decentralized storage solutions
- Real-time blockchain analytics
Trusted by industry leaders and our families — because bribery is our love language.
Frequently Asked Questions
If you can’t find what you’re looking for, email our support team and if you’re lucky someone will get back to you.
How does end-to-end encryption work on a decentralized network?
End-to-end encryption in a Web3 environment ensures that only the sender and recipient can access messages or transactions. Your data is encrypted on your device and can only be decrypted by the intended recipient, with no central authority having access, making it truly private and censorship-resistant.
What happens if I lose my private key?
Losing your private key means losing access to your encrypted data and wallet. Since Web3 platforms are decentralized, there is no central authority to recover it. We recommend securely backing up your key using a hardware wallet or a non-custodial solution.
Are self-destructing messages truly permanent?
Self-destructing messages are removed from decentralized storage after the specified time. However, like any system, recipients may still take screenshots or record content, so always use discretion when sharing sensitive information.
Eager to become a partner?
Sign up here. Our team will promptly get in touch with you, providing an enablement pack tailored to your specific requirements.